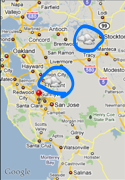
前回の続き:
[iPhone] Map Kitで遊ぼう:地図を出して現在位置にピンを立てる
http://blog.s21g.com/articles/1596
参考:
Drawing polyines or routes on a MKMapView (as an MKAnnotationView) – Part 2
http://spitzkoff.com/craig/?p=108
MyAnnotation.h
1
2
3
4 @interface MyAnnotation : NSObject <MKAnnotation> {
5 CLLocationCoordinate2D _coordinate;
6 }
7
8 @property (nonatomic, readonly) CLLocationCoordinate2D coordinate;
9
10 -(id)initWithCoordinate:(CLLocationCoordinate2D) coordinate;
11
12 @end
MyAnnotation.m
1
2
3 @implementation MyAnnotation
4
5 @synthesize coordinate=_coordinate;
6
7 -(id)initWithCoordinate:(CLLocationCoordinate2D) c{
8 _coordinate=c;
9 return self;
10 }
11
12 @end
ポイントとしては
- .hでMKMapViewDelegateを実装宣言
- _mapView.delegate = self;
- -(MKAnnotationView )mapView:(MKMapView )mapView viewForAnnotation:(id )annotationを実装
- MyAnnotationなら画像を設定する:
if([annotation isKindOfClass:[MyAnnotation class]]) {
annotationView.image = [UIImage imageNamed:@"some.png"];
本当はMKAnnotationViewを派生して、MyAnnotationViewを作るのがベターなんですけど省略しています。
MapTutorialViewController.h
1
2
3
4 @interface MapTutorialViewController : UIViewController <MKMapViewDelegate> {
5 MKMapView *_mapView;
6 }
7 @end
MapTutorialViewController.m
1
2 - (MKAnnotationView *)mapView:(MKMapView *)mapView viewForAnnotation:(id <MKAnnotation>)annotation{
3 MKAnnotationView *annotationView;
4 NSString* identifier = @"Pin";
5 annotationView = (MKPinAnnotationView*)[_mapView dequeueReusableAnnotationViewWithIdentifier:identifier];
6 if(nil == annotationView) {
7 annotationView = [[[MKPinAnnotationView alloc] initWithAnnotation:annotation reuseIdentifier:identifier] autorelease];
8 }
9 if([annotation isKindOfClass:[MyAnnotation class]]) {
10 annotationView.image = [UIImage imageNamed:@"some.png"]; //地図に表示したい画像を設定
11 }
12 return annotationView;
13 }
14
15 - (void)addSomeAnnotations {
16 //a location
17 double latitude = 37.331688999999997;
18 double longitude = -122.030731;
19 CLLocationCoordinate2D _location;
20 _location.latitude = latitude;
21 _location.longitude = longitude;
22
23 CLLocationCoordinate2D location1;
24 MyAnnotation *annotation;
25
26 location1.latitude = _location.latitude+0.1;
27 location1.longitude = _location.longitude+0.1;
28 annotation =[[MyAnnotation alloc] initWithCoordinate:location1];
29 [_mapView addAnnotation:annotation];
30
31 location1.latitude = _location.latitude+0.5;
32 location1.longitude = _location.longitude+0.5;
33 annotation =[[MyAnnotation alloc] initWithCoordinate:location1];
34 [_mapView addAnnotation:annotation];
35 }
36
37 - (void)viewDidLoad {
38 [super viewDidLoad];
39
40 _mapView = [[MKMapView alloc] initWithFrame:self.view.bounds];
41 _mapView.showsUserLocation=TRUE;
42 _mapView.delegate = self;
43 [self.view addSubview:_mapView];
44
45 [self addSomeAnnotations];
46
47 }
48